Agario like
This initial exercise for my school was to create an notes manager with React, Express and Mongo DB. But I have alredy done this kind of project and i find it a lit bit (a lot) boring. The other option was to create an agario like game with multiplayer. But It's boring, less boring than the first option but boring. So I decided to do it in 3D, that didn't change the game but it was more cool and sexy.
Technologies
- Express
- Socket.io
- React
- R3F
- tailwindcss
- Rapier
What We need
A board
A moving player
Some food
Multiplayer
What I want
Some obstacles
A leaderboard
Server socket
It manages everything from board configuration to player position and collisions (except for obstacles).
To begin with, it generates a map:
- random generation of obstacles in map space
- food generation where there are neither obstacles nor food
- waiting for players to connect
When a player connects, it will send him the config, as well as food on the map, obstacles and players already connected.
He'll also add it to players with an initial position that means it won't spawn on a player, obstacle or food.
When the player moves, we check for collisions (we should also make an anti-cheat to calculate the player's velocity and make sure he doesn't make instantaneous moves, but I haven't had time to integrate it).
If a player has a collision with food, we increase his size by 1 and his score by 1 (both have the same value because linear growth was requested, but in reality we should apply this kind of function :
$$ f(x) = k - (k - b)e^{-ax} $$Where k is the maximum size of the player, where b is the minimum size of the player and where a is a coeficient that will “smooth” the curve, to avoid a linear progression and allow new players to have a chance of catching up with number 1). Player speed is linear, but should be calculated with a function like :
$$ f(x) = b+(k-b)e^{-ax} $$If the player has a collision with another player, we take the score of the eaten player and add it to the score of the player who has eaten (we also make a comparison to see who has a higher score and therefore eats the other player).
Client
The first step was to create a context to manage all our interactions. It manages the reception of different events:
- connect: when the player establishes a connection with the socket server
- initialize: receives initial game data
- playerMoved: when a player moves
- playerJoined: when a player joins the game
- playerLeft: when a player leaves the game
- updateFoods: when the food is updated (in our case a bit duplicative with the next one, but with a view to future improvements it could be very interesting)
- foodEaten: food is eaten
- playerEaten: a player is eaten
- playerUpdated: when our own player is updated
Each time a player moves, we round up its position to avoid spamming the server. If it's different, we send it to the server, then all the other players pick up the new position.
When the server informs us that the player has eaten, we change his size and hit box, which is calculated as follows:
One of the problems of making a hitbox the size of the player is that if another, smaller player hides in a corner of the field, the larger player will never be able to reach the player.
The beam must be allowed to reach the angles: in other words, calculate A
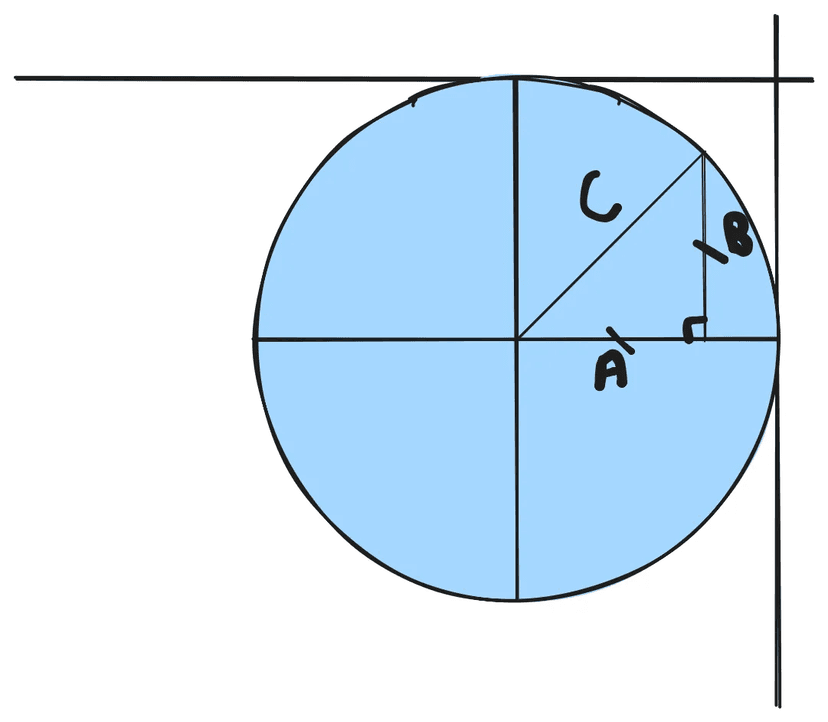
We know that A = B and we know our radius, so C
$$ C^2=2A^2 $$$$ A=\sqrt{\frac {C^2} 2} $$Then we generate the cylinders for the food according to our context.
For other players too.
And the same goes for obstacles, except that we tell them they're rigid so that they have physics, and we also add a restitution coef of less than 1 to avoid infinite bounces.